Hi guys,
I am working on a custom "ContactFieldConverter" [the thing that splits the Contact's FullName imported by excel/ webserveces etc] in order to split new contact's names into "GivenName" and "Surname", and preventing the OOTB function from filling in the "MiddleName" column.
In order to do so, I created a custom ContactGsFieldConverter in my package and inserted the value of the Separator and Converter in the Lookup "ShowNamesBy" and sysSetting "ContactFieldConverter".
[myContactGsFieldConverter code:]
namespace Terrasoft.Configuration {
using System;
using System.Linq;
using System.Text;
using Terrasoft.Common;
using Terrasoft.Configuration;
#region Class: myContactGsFieldConverter
/// <summary>
/// Contact "Full name" field converter class.
/// Separates "Full name" string using "Given name Surname" rule.
/// </summary>
public class myContactGsFieldConverter : IContactFieldConverter
{
#region Properties: Public
/// <summary>
/// Contact "Full name" separator characters array.
/// </summary>
private char[] _separator = { ' ' };
public char[] Separator {
get {
return _separator;
}
set {
_separator = value;
}
}
#endregion
#region Methdos: Public
/// <summary>
/// <see cref="IContactFieldConverter.GetContactSgm"/>
/// </summary>
/// <remarks>
/// After splitting <paramref name="name"/> first element will be used as <see cref="ContactSgm.GivenName"/>,
/// Everything else as <see cref="ContactSgm.Surname"/>.
/// </remarks>
ContactSgm IContactFieldConverter.GetContactSgm(string name) {
var sgm = new ContactSgm();
if (string.IsNullOrEmpty(name)) {
return sgm;
}
var array = name.Split(Separator, StringSplitOptions.RemoveEmptyEntries);
switch (array.Length) {
case 0:
return sgm;
case 1:
sgm.GivenName = array[0];
break;
case 2:
sgm.GivenName = array[0];
sgm.Surname = array[1];
break;
default:
sgm.GivenName = array[0];
StringBuilder sb = new StringBuilder();
for (var i = 1; i <= array.Length - 1; i++) {
sb.AppendFormat("{0} ", array[i]);
}
sgm.Surname = sb.ToString().Trim();
break;
}
return sgm;
}
/// <summary>
/// <see cref="IContactFieldConverter.GetContactName"/>
/// </summary>
/// <remarks>
/// "Full name" string will be created using "Given name [Middle name] Surname" rule.
/// </remarks>
public string GetContactName(ContactSgm sgm) {
var concatChar = Separator.FirstOrDefault();
return new[] { sgm.GivenName, sgm.MiddleName, sgm.Surname }.ConcatIfNotEmpty(concatChar.ToString());
}
#endregion
}
#endregion
}
The converter is not working. I tried to debug and noticed that my contact converter is returned as null to [this is a method in the Contact c# object]:
public virtual void SetSgm(Contact contact) {
if (contact == null) {
return;
}
contact.FillSgmFields(GetContactConverter());
}
Do you know if I need to do something specific in case of separate-assembly package?
ie: Using a different namespace for the converter/ setting the converter path in a different way within the system setting/ recreate the method on the Contact Object in my custom package.

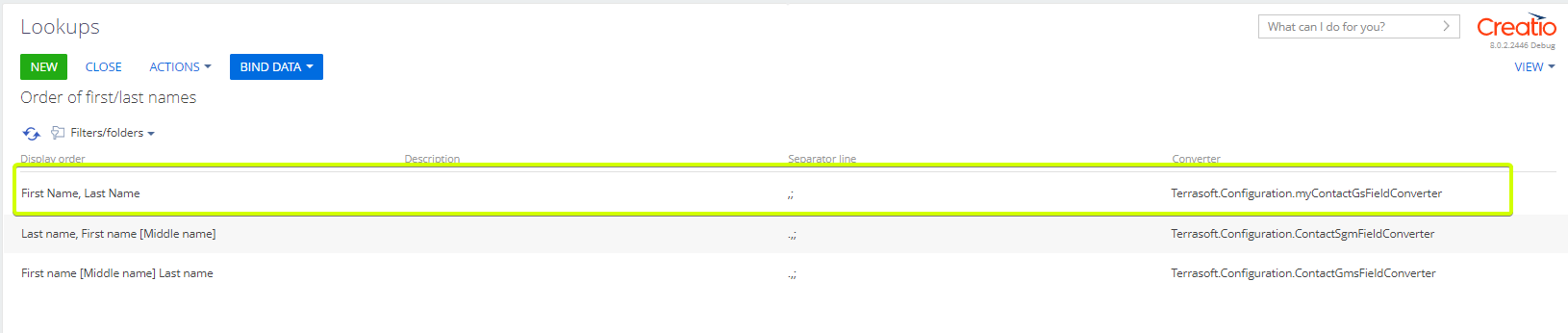
Thank you in advance!