I have created a signal-based Business Process that fires on modifying the data in Opportunity. 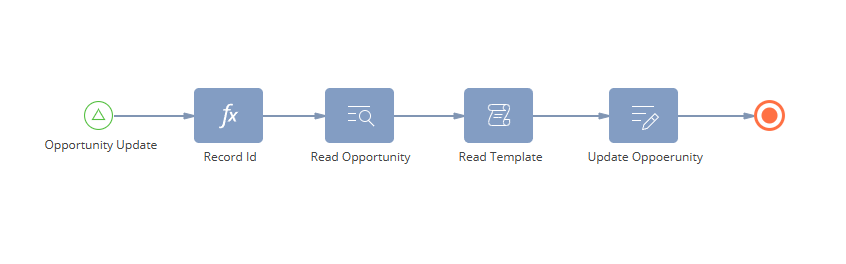
To Read the Email Template I write the following code:
using System;
using System.Collections.Generic;
using System.Linq;
using Newtonsoft.Json;
using Terrasoft.Common;
using Terrasoft.Configuration;
using Terrasoft.Configuration.Utils;
using Terrasoft.Core;
using Terrasoft.Core.Configuration;
using Terrasoft.Core.DB;
using Terrasoft.Core.Entities;
using Terrasoft.Core.OperationLog;
using SystemSettings = Terrasoft.Core.Configuration.SysSettings;
public class EmailTemplateManager
{
private UserConnection _userConnection;
private MacrosHelperService _macrosService;
private readonly Dictionary<Guid, Entity> _cachedTemplates = new Dictionary<Guid, Entity>();
public EmailTemplateManager(UserConnection userConnection) : base() {
_userConnection = userConnection;
MacrosHelperV2 macrosHelper = new GlobalMacrosHelper();
macrosHelper.UserConnection = userConnection;
_macrosService = new MacrosHelperService(macrosHelper, userConnection);
}
public string GetEmailTemplateSchemaName() {
return _userConnection.GetFeatureState("EmailMessageMultiLanguage") == 0
? "EmailTemplate"
: "EmailTemplateLang";
}
public string GetTemplateBody(Guid recordId, Guid templateId) {
var template = GetTemplate(templateId);
if(template != null) {
var schemaName = GetSchemaNameFromId(template.GetTypedColumnValue<Guid>("ObjectId"));
var body = this.GetTemplateBody(new MacrosHelperServiceRequest
{
EntityId = recordId,
EntityName = schemaName,
TemplateId = templateId
});
return body;
}
return string.Empty;
}
public string GetTemplateBody(MacrosHelperServiceRequest request) {
MacrosHelperServiceResponse template = null;
string body = string.Empty;
if (_userConnection.GetIsFeatureEnabled("EmailMessageMultiLanguageV2")) {
template = _macrosService.GetMultiLanguageTextTemplate(request);
body = template.TextTemplate;
} else {
template = _macrosService.GetMultiLanguageTextTemplate(request);
body = template.TextTemplate;
}
return body;
}
public string GetTemplateSubject(MacrosHelperServiceRequest request) {
MacrosHelperServiceResponse template = _macrosService.GetMultiLanguageTextTemplate(request);
string title = template.SubjectTemplate;
return title;
}
private bool IsNeedChangeMacrosCulture() {
return _userConnection.GetIsFeatureEnabled("UseMacrosAdditionalParameters")
&& _userConnection.GetIsFeatureEnabled("EmailMessageMultiLanguageV2");
}
public string GetTemplateSubject(Guid recordId, Guid templateId) {
var template = GetTemplate(templateId);
if(template != null) {
var schemaName = GetSchemaNameFromId(template.GetTypedColumnValue<Guid>("ObjectId"));
return GetTemplateSubject(new MacrosHelperServiceRequest
{
EntityId = recordId,
EntityName = schemaName,
TemplateId = templateId
});
}
return string.Empty;
}
private string GetSchemaNameFromId(Guid objectId) {
if(objectId != Guid.Empty) {
var selectQuery = new Select(_userConnection)
.Column("Name")
.From("SysSchema")
.Where("UId")
.IsEqual(Column.Parameter(objectId)) as Select;
return selectQuery.ExecuteScalar<string>();
}
return string.Empty;
}
public Entity GetTemplate(Guid id) {
Entity template;
if (_cachedTemplates.ContainsKey(id)) {
template = _cachedTemplates[id];
} else {
var esq = new EntitySchemaQuery(_userConnection.EntitySchemaManager, GetEmailTemplateSchemaName());
esq.AddColumn("Object");
esq.AddColumn("Subject");
esq.AddColumn("Body");
esq.Filters.Add(esq.CreateFilterWithParameters(FilterComparisonType.Equal, "Id", id));
template = esq.GetEntity(_userConnection, id);
_cachedTemplates.Add(id, template);
}
return template;
}
}
To call this class in the Business Process I used this code:
try{
var userConnection = GetUserConnection();
var emailManager = new EmailTemplateManager(userConnection);
var templateId = Get<Guid>("TemplateId");
var recordId = Get<Guid>("RecordId");
var subject = emailManager.GetTemplateSubject(recordId, templateId);
var body = emailManager.GetTemplateBody(recordId, templateId);
Set<String>("Body", body);
Set<String>("Subject", subject);
}
catch(Exception ex){
Set<String>("Subject", ex.Message);
Set<String>("Body", ex.StackTrace);
}
return true;
To get the UserConnection in the background I used this Code:
private UserConnection GetUserConnection(){
return Get<UserConnection>("UserConnection");
}
Here is the error I get:
Error Message: Error creating an instance of the "Terrasoft.Configuration.ILanguageIterator" class
Stack Trace: at Terrasoft.Core.Factories.ClassFactory.GetInstance[T](Func`1 action)
at Terrasoft.Configuration.MLangContentFactory.GetContentKit(String iteratorTagName, String storeTagName)
at QTECX.Email.EmailTemplateManager.GetTemplateSubject(String schemaName, Guid recordId, Guid tplId)
at QTECX.Email.EmailTemplateManager.GetTemplateSubject(Guid recordId, Guid templateId)
at Terrasoft.Core.Process.QTXTestEmailProcessMethodsWrapper.ScriptTask1Execute(ProcessExecutingContext context)